Sometimes in Powershell the Column label names can be not very intuitive or you may have to present the results to a non technical person who may not understand. In this case we can edit the labels to suit our needs.
The format is quite simple we will use Get-Process in this example. When filtering the property you wish to change the label of use the following code:
@{} Use @ and all your code for the custom column goes between the curly brackets.
Inside of the curly brackets insert the following:
Label="Custom column Label";
Expression={$_.Property Name}
And all together it will look like below.
Get-Process | Format-Table Name,ID,@{Label="Paged Memory Size";Expression={$_.PM }} -AutoSize
You can also further format the output so it better suits your needs. Here we will use Get-WmiObject to see available disk space and format the column header and the output to show us how many GB of free space we have to 2 decimal places.
Here we nee to add some extra code to get the outcome we desire.
/1GB We have divided the $_.freespace property by 1GB you can also divide it by MB,TB and PB if you desire.
formatstring="N2" restricts the output to 2 decimal places you just need to change the number after the N if you desire more or less decimal places.
width=20 Specifies the column header width.
align="Left" Sets the alignment of the data within the column.
All together it looks like:
@{Name="FreeSpaceGB";Expression{$_.freespace /1GB};formatstring="N2";width=20;align="left"}
And the full script will look like:
Get-WmiObject Win32_LogicalDisk -Filter DriveType=3 | Format-Table SystemName,VolumeName,DeviceId,@{Name="Free Space GB";Expression={$_.freespace / 1GB};formatstring="N2";width=20;align="left"}
Test Email Via Telnet
Often you will need to test if email is working correctly within your organization. and a great way to do that is through Telnet.
First you will need to know the host name or ip address of the mail server or relay you wish to test.
If you don't know you can use nslookup.
Use Nslookup to determine your mail server.
1. Open an Administrative command prompt.
2. Type "nslookup" press enter.
3. Type "set type=mx" press enter.
4. Type your domain name.
5. It will then return results showing server name and ipaddress.
Now you have the mail server we can make the Telent connection.
1. Open an administrative Command prompt window.
2 Type "telnet YourMailServer 25" press enter.
3. Type "ehlo yourdomain.com" press enter.
4. Type "mail from:youremail@yourdomain.com" press enter.
5. Type "rcpt to:recpient@yourdomain.com" press enter.
6. Type "data" press enter.
7. Type a message(email body) eg. "this is a test" press enter
8. Type "." press enter. This tells the server you are finished with the body of the message and to go ahead and send the email.
9. Type "Quit" to exit the telnet session.
You have now sent a test email message via Telent!
First you will need to know the host name or ip address of the mail server or relay you wish to test.
If you don't know you can use nslookup.
Use Nslookup to determine your mail server.
1. Open an Administrative command prompt.
2. Type "nslookup" press enter.
3. Type "set type=mx" press enter.
4. Type your domain name.
5. It will then return results showing server name and ipaddress.
Now you have the mail server we can make the Telent connection.
1. Open an administrative Command prompt window.
2 Type "telnet YourMailServer 25" press enter.
3. Type "ehlo yourdomain.com" press enter.
4. Type "mail from:youremail@yourdomain.com" press enter.
5. Type "rcpt to:recpient@yourdomain.com" press enter.
6. Type "data" press enter.
7. Type a message(email body) eg. "this is a test" press enter
8. Type "." press enter. This tells the server you are finished with the body of the message and to go ahead and send the email.
9. Type "Quit" to exit the telnet session.
You have now sent a test email message via Telent!
Add user to local Computer group via Comand Line
You can add users to a local security group via the command line with the following command:
netlocalgroup "groupname" "username" /add
and to confirm it worked:
netlocalgroup "groupname"
I wrote a simple little script to run this via powershell with user input.
If you have the psexec tools installed you can run it for a remote machine using the following command
psexec \\RemotePC -u UserName -p password net localgroup "Localgroup" "Username" /add
You can download PsExec tools from here.
netlocalgroup "groupname" "username" /add
and to confirm it worked:
netlocalgroup "groupname"
I wrote a simple little script to run this via powershell with user input.
If you have the psexec tools installed you can run it for a remote machine using the following command
psexec \\RemotePC -u UserName -p password net localgroup "Localgroup" "Username" /add
You can download PsExec tools from here.
Could not flush the DNS Resolver cache: Function Failed During Execution. When flushing DNS cache
To resolve this you need to restart the DNS Client service. You can do this by opening the Services management console by selecting windows button+r and typing services.msc, and then within the console restarting the DNS Client Service or starting it if it's turned off. Ensure the service is running and the startup type is set to automatic after you do so.
You can also achieve the same task through the command prompt by typing:
net stop "dns client" and then
net start "dns client"
Then you are done and will be able to successfully flush the dns cache on the machine.
Compare Active Directory Group Membership with Powershell
Here is a nice little Powershell script I wrote to compare group membership of two active directory users. You can use this script for many different purposes just edit the reference command and property to whatever object you wish to compare.
$UserReference = Get-ADPrincipalGroupmembership (Read-Host "Reference User")
$UserDifference = Get-ADPrincipalGroupmembership (Read-Host "Difference User")
Compare-object $UserReference $UserDifference -property SamAccountName
=> Means the Difference User is a member of a group and the Reference User is not a member.
<= Means the Reference User is a member of the group and the Difference User is not a member.
You can also use the -IncludeEqual parameter to include groups both users are a member of that will be represented by the SideIndicator value of: ==
$UserReference = Get-ADPrincipalGroupmembership (Read-Host "Reference User")
$UserDifference = Get-ADPrincipalGroupmembership (Read-Host "Difference User")
Compare-object $UserReference $UserDifference -property SamAccountName
=> Means the Difference User is a member of a group and the Reference User is not a member.
<= Means the Reference User is a member of the group and the Difference User is not a member.
You can also use the -IncludeEqual parameter to include groups both users are a member of that will be represented by the SideIndicator value of: ==
RE Create Profile in Windows 7
To re create a user profile in windows 7 you must remove the profile from the registry otherwise the user will get a temp profile when they log in next.
To re create the profile you first have to re name the user folder in c:\users so you can retrieve the user data when they login to the new profile
Then you have to delete the profile in Advanced system properties by going to:
control panel>system and security>system
or
Right click computer and select properties
Select the "Advanced system settings" option
In the "User Profiles" field select "Settings"
Then select the profile you would like to remove and select "Delete"
Then open the registry editor by selecting windows button + R and typing regedit and browse to: HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\ProfileList
Select the profile you want to remove right click it and select "delete"
Restart the computer and get the user to log in to create their new profile and copy the users files over form the renamed folder.
Disable Server Manager on logon with Group Policiy
1. Select "Start," "Administrative Tools"
and "Group Policy Management."
2. Navigate to the "Local Computer Policy/Computer
Configuration/Administrative Templates/System/Server Manager/" sub-folder
in the left pane of the Group Policy Management window.
3.Double-click the "Do Not Display Server Manager
Automatically at Logon" setting in the right pane.
4. Select "Enabled" and then Select "OK."
5. Link the GPO to the appropriate OU that contains your servers and you are done.
Log off user session on remote machine using Powershell
This is a handy little tool you can use with Powershell or in command prompt to see who is logged onto a remote machine and to end there session. Great for servers when you get the "too many users already logged in" message when trying to access a server.
1. You need to get the session ID of a user to log off by running the following command:
2. Enter the following command with the session ID at the end to log the user of:
1. You need to get the session ID of a user to log off by running the following command:
qwinsta /server:servername
2. Enter the following command with the session ID at the end to log the user of:
logoff /server:servername (session id)
I used these commands to put together the following script. It will prompt you for the server name and give you the output of current open sessions then prompt you for the session ID to log off. Enjoy:
$server = read-host "Hostname"
qwinsta /server:$server
$ID = read-host "Session ID to log off"
logoff /server:$server $ID
Group Policy: Create mapped drive that is synced locally to be available offline.
If you have users that go to client sites and will not always have access to a network connection, and need access to some non confidential documents on the network creating Administratively assigned cashed files can be the way to go.
2. In Group policy Management Editor expand:
User configuration> Administrative Templates> Network>
Offline Files> Select Specify Administratively assigned Offline Files
3. Select Enabled.
Then Select "Show"
In the show contents window enter the name of the UNC path of the network share in the "Value Name" field. Leave the value field blank and select ok. Creating the offline share is done.
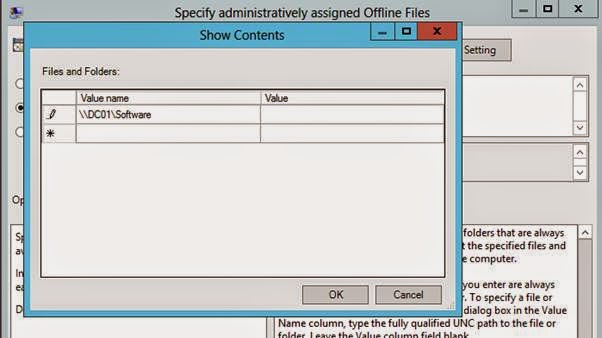
And in the same policy we will map the drive for the share that we just made available offline.
4. Again in Group Policy Management Editor Expand:
Preferences> Windows Settings> Drive Maps
1. Set Action to "Update" (This will re-map the drive if the user removes it)
Set Location: the location of the network share.
Select the "Reconnect" radio button
Label as: Gives the share a desired name
Select a drive letter
select apply
Link the GPO to the desired organizational unit that contains the users you wish to provide the offline files to. When they log in next they will have a mapped drive that will sync locally and be available to use offline.
1. Create a new group policy object.
2. In Group policy Management Editor expand:
User configuration> Administrative Templates> Network>
Offline Files> Select Specify Administratively assigned Offline Files
3. Select Enabled.
Then Select "Show"
In the show contents window enter the name of the UNC path of the network share in the "Value Name" field. Leave the value field blank and select ok. Creating the offline share is done.
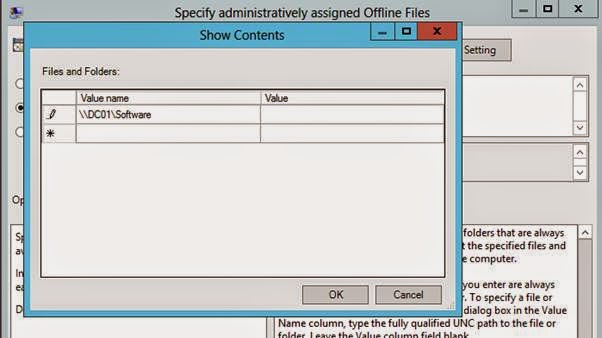
And in the same policy we will map the drive for the share that we just made available offline.
4. Again in Group Policy Management Editor Expand:
Preferences> Windows Settings> Drive Maps
1. Set Action to "Update" (This will re-map the drive if the user removes it)
Set Location: the location of the network share.
Select the "Reconnect" radio button
Label as: Gives the share a desired name
Select a drive letter
select apply
Link the GPO to the desired organizational unit that contains the users you wish to provide the offline files to. When they log in next they will have a mapped drive that will sync locally and be available to use offline.
Powershell: Reset user password and set to change at next logon
This powershell script can be used to reset user passwords quickly and force them to change it on their next logon. Copy this script into powerhell ISE and save it as a .ps1 file. When you run the script it will prompt you for the username. You can change the password variable to be whatever you want.
$Identity = read-host "Enter User name"
$password = P455w0rd
Set-adaccountpassword -Identity $Identity -reset -newpassword (ConvertTo-SecureString -AsPlainText $password -Force)
Set-aduser $user -changepasswordatlogon $true
or to statically assign the password use the following script:
Set-adaccountpassword -Identity (read-host "Enter User name") -reset
-NewPassword (Read-Host -AsSecureString "New Password")
$Identity = read-host "Enter User name"
$password = P455w0rd
Set-adaccountpassword -Identity $Identity -reset -newpassword (ConvertTo-SecureString -AsPlainText $password -Force)
Set-aduser $user -changepasswordatlogon $true
or to statically assign the password use the following script:
Set-adaccountpassword -Identity (read-host "Enter User name") -reset
-NewPassword (Read-Host -AsSecureString "New Password")
Format Hard Disk or USB Removable Storage using Diskpart
Format Hard Disks or USB removable storage using the
diskpart command line utility.
Open command prompt
Diskpart – To initiate the diskpart utility
List disk – To list all available disks.
Select disk – Select the disk you wish to format.
Detail disk – Find out more details about the selected disk. Handy to make sure you are formatting the right disk.
Clean all – Formats the disk
Create partition primary – Creates a partition on the disk.
If you don’t specify the size all available space will be used by default.
Select partition 1 - Select desired partition
Active – Sets the selected partition as active.
Format fs=(enter desired filesystem ntfs, fat32 ect.) –
formats the volume with the requires file system
Assign – to give the drive the next available drive letter
or you can specify one by typing
Assign letter=(desired letter)
Exit – to exit diskpart
Restore a Deleted Active Directory Object
To restore a deleted Active Directory Object in Server 2008 or 2003 (this can also be preformed also in server 2012 but the active directory recycle bin is much easier and faster) you can perform a using ldp.exe to recover the items as long as the time to live for the deleted objects container hasn't passed.
1. Log in to Active Directory Server as an Domain Administrator
2. Open ldp.exe using Command Prompt or Powershell

3. Select Connection menu, select Connect, then type server name.
4. Select Connection menu, select Bind and Select OK
The Deleted Objects folder is hidden by default you will need to make it visible
5. Select Options menu and Select Controls.
Expand the Load Predefined container list and select Return deletedobjects. Select OK
6. Select the View menu, select Tree, and then use the dropdown to select the distinguished name of the domain. For Example DC=Contoso,DC=com.
7. Expand the Domain Name container and then Expand Deleted Objects container.
8. Right click on the desired account, then click Modify.
9. In the Attribute box, type isDeleted. Under Operation, select Delete, and then select Enter.
10. In the Attribute box, type distinguishedName, in the Values box, type:
CN=User Name,OU= Organizational Unit,DC=domain,DC=name.
Under operation, select Replace and then Select Enter.
11. Select the Extended check box and then Select Run.
You will need to reset password, enable the account and fill in any missing attributes and groups
Done! It is much easier with server 2012 and the active directory recycle bin. But if using server 2008
or below this is the easiest and least disruptive way to restore a deleted user account.
View Applied Group Policy for Logged on User
To troubleshoot issues relating to group policy run one of the following commands.
To generate a report in html format type into Command Prompt or Powershell:
Gpresult /h c:\test.html(or whatever local or network location you desire)
Then browse to the location and open the report in a web browser.
To view the results within Command Prompt or Powershell simply type:
Find who is logged onto a computer within domain
Someone causing havoc on your network and all you have is the computername? Here is a handy little powershell script to find the culprit.
1. Open Powershell
2. Enter the following script.
1. Open Powershell
2. Enter the following script.
Remove Driver Package from Driver Store
Handy little command line tool to permanently delete drivers from the driver store in Windows.
1. Open a administrative command prompt
2. to view drivers in driver store type:
pnputil.exe -e
3. Find the driver you want to remove and insert the "Published Name" into the command
1. Open a administrative command prompt
2. to view drivers in driver store type:
pnputil.exe -e
3. Find the driver you want to remove and insert the "Published Name" into the command
pnputil.exe -d oem$.inf
$=number of the drivers Published Name.
4. Done.
Adding Driver package to Windows installation
You can add your own driver package to a bootable windows installation using dism. It can save a lot of time and heartache post installation.
1. First I like to move the install.wim file to an accessable location. On the windows installation media open the sources folder and copy the install.wim file to your root directory.
2. Then create a folder in your root directory in this case I like to use "mount"
3. Open an administrative command prompt
3. To mount the wim file type:
dism.exe /Mount-WIM /WimFile:"C:\install.wim" /index:1 /MountDir:"C:\mount"
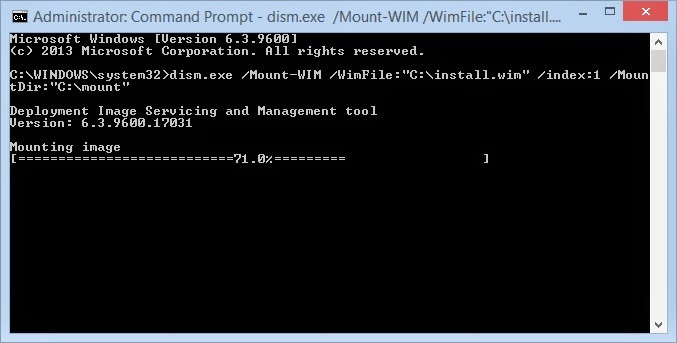
4. To inject the drivers into the wim type:
dism /image:C:\mount /Add-Driver /driver:"C:\Driver location" /recurse
(ensure all drivers are located in the same location and the packages have been unzipped to expose the files)
dism /image:C:\mount /Add-Driver /driver:"C:\Driver location" /recurse
(ensure all drivers are located in the same location and the packages have been unzipped to expose the files)
5. To unmount and commit the changes type:
dism /unmount-wim /Mountdir:C:\mount /commit
6. Copy the wim file back into sources folder on the windows installation media.
7. Done! Never worry about about drivers again.
Give Calendar permissions with Powershell
Here is a handy Powershell script I wrote to give Calendar permissions via Powershell. Paste the script into Powershell ISE and play it. We often get requests from PA's and senior staff needing fast access to users calendars this makes short work of it. It will add the exchange snap-in, then prompt you for the users mailbox. Enter the user whose calendar is being granted access to. Then the current calendar permissions will be shown then you will be prompted to enter the user requiring access. Then enter permission level and the script will finally display the permissions after the change. (This is for Exchange 2010 for other versions remove the snapin command from the first line.)
add-pssnapin Microsoft.Exchange.Management.PowerShell.E2010
cls
$user = $null
$user = read-host "Calendar Being Configured"
$user = $user + ":\calendar"
get-mailboxfolderpermission -Identity $user | ft user,accessrights
$Identity = $null
$Identity = read-host "User Being Granted Access"
$AccessRights = $null
$AccessRights = read-host "Access Required - Editor,Owner,Reviewer,Contributor"
Add-MailboxFolderPermission -Identity $User –User $Identity –AccessRights $AccessRights
get-mailboxfolderpermission -Identity $user | ft user,accessrights
Subscribe to:
Posts (Atom)